I had a puzzle recently with a RadComboBox...
I have a sql query that took a long time to run... sometimes 30 seconds, due to the huge database size and the complex query...
To populate a RadGrid - I bound it to a SqlDataSource. But I also needed dropdowns to filter this grid - and this needed to be bound to unique items in a column of that grid... I didnt want to do another db query to get a filtered version of the same info - so I bound it to the SAME SqlDataSource - and cached the datasource to minimize calls.
[asp:SqlDataSource ID="MySqlDataSource" EnableCaching="true" CacheDuration="180"
runat="server" OnSelecting="MySqlDataSource_Selecting"
ConnectionString="[%$ ConnectionStrings:mySqlConnectionString %]"
SelectCommand="SELECT * FROM vwBundlesForSale "]
[/asp:SqlDataSource]
However, this made there to be duplicate items in my dropdown... and the data was not sorted.
If you want to Sort and Filter for unique items in a Telerik RadComboBox, use something like this:
IN ASPX PAGE:
[telerik:RadComboBox ID="CoatComboBox"
runat="server" DataSourceID="MySqlDataSource" DataTextField="CoatName" DataValueField="CoatName" AllowCustomText="false" EnableItemCaching="true" Width="165px" DropDownWidth="260px" OnDataBound="SortAndNoDups_OnDataBound" AppendDataBoundItems="true" ......
IN CS CODE-BEHIND
protected void SortAndNoDups_OnDataBound(object sender, EventArgs e)
{
RadComboBox fixComboBox = (RadComboBox)sender;
string selectedText = string.Empty;
if (fixComboBox.SelectedIndex >= 0)
{
selectedText = fixComboBox.SelectedValue;
}
fixComboBox.SelectedIndex = -1;
fixComboBox.Filter = RadComboBoxFilter.Contains;
fixComboBox.Sort = RadComboBoxSort.Ascending;
fixComboBox.SortItems();
string priorItem = "";
for (int i = 0; i < fixComboBox.Items.Count; i++)
{
try
{
RadComboBoxItem itm = fixComboBox.Items[i];
if (itm.Text == priorItem)
{
fixComboBox.Items.Remove(itm);
i--;
}
else
{
priorItem = itm.Text;
}
}
catch
{
break;
}
}
if (selectedText != string.Empty)
{
try
{
fixComboBox.SelectedValue = selectedText;
}
catch { }
}
}
protected void MySqlDataSource_Selecting(object sender, SqlDataSourceSelectingEventArgs e)
{
e.Command.CommandTimeout = 0; //prevents SqlDataSource timeout after 30 seconds
}
Sunday, December 12, 2010
Wednesday, November 10, 2010
C# ?? two question marks
You ever wonder what ?? means in C# language?
Its a cool shortcut for checking for nulls and returning a value...
I find it very useful when working with Linq queries... that may return some nullable fields..
Great article:
http://stackoverflow.com/questions/446835/what-do-two-question-marks-together-mean-in-c
Its a cool shortcut for checking for nulls and returning a value...
I find it very useful when working with Linq queries... that may return some nullable fields..
Great article:
http://stackoverflow.com/questions/446835/what-do-two-question-marks-together-mean-in-c
Tuesday, September 7, 2010
Soap Call using Javascript
There are many times now that I need to make a soap call to an XML web service.... from the client (javascript). If, for example, you need to update the database... but want to use Javascript to do so out of process - without a postback...and with minimal data passed to the server... this is a great approach.
After a lot of research and testing I have found and do recommend the following js tool for any of my colleagues:
http://www.codeproject.com/KB/ajax/JavaScriptSOAPClient.aspx
Using this, it is a simple trick to call the database...
[script]
var url = "../../ws/MyWebService.asmx";
function HelloWorld() {
var pl = new SOAPClientParameters();
pl.add("yourName", "Robert the Great");
SOAPClient.invoke(url, "HelloTo", pl, true, Hello_CallBack);
}
function Hello_CallBack(resultData) {
alert("Hello_CallBack: " + resultData);
}
[/script]
After a lot of research and testing I have found and do recommend the following js tool for any of my colleagues:
http://www.codeproject.com/KB/ajax/JavaScriptSOAPClient.aspx
Using this, it is a simple trick to call the database...
[script]
var url = "../../ws/MyWebService.asmx";
function HelloWorld() {
var pl = new SOAPClientParameters();
pl.add("yourName", "Robert the Great");
SOAPClient.invoke(url, "HelloTo", pl, true, Hello_CallBack);
}
function Hello_CallBack(resultData) {
alert("Hello_CallBack: " + resultData);
}
[/script]
Sunday, July 25, 2010
Optimizing a website for Speed
There are several techniques that can be used to optimize your website for speed. And remember, one of the things Google considers now is your website speed.
The basics include
1) Compressing resource files (like .css and js)
2) Minimizing the number of files linked/imbedded in a web page.
3) Optimizing the size of images used on the web page
NOTE: if a css file referenced on all pages references multiple images for backgrounds - make sure that most of them are really used on all pages. If not - consider referencing them only on pages where needed.
So for my websites, I am taking all the common css files for a page (often separated for specific wigits or sections) and compressing them into one .css file for the site. I do the same for the javascript... Of course, this is only after the site is approved for look/feel by the client so I don't have to redo this. And I keep the un-compressed versions available for later editing if needed... since these will have all my comments and easy-to-read formatting.
If a .css or .js file is only needed on a few pages, I only include a compressed version of that file where needed. (and I use the compressed version here too...) But overall, this has proved to be a good strategy for minimizing website overhead - and increasing speed.
TOOLS TO BOOKMARK:
The basics include
1) Compressing resource files (like .css and js)
2) Minimizing the number of files linked/imbedded in a web page.
3) Optimizing the size of images used on the web page
NOTE: if a css file referenced on all pages references multiple images for backgrounds - make sure that most of them are really used on all pages. If not - consider referencing them only on pages where needed.
So for my websites, I am taking all the common css files for a page (often separated for specific wigits or sections) and compressing them into one .css file for the site. I do the same for the javascript... Of course, this is only after the site is approved for look/feel by the client so I don't have to redo this. And I keep the un-compressed versions available for later editing if needed... since these will have all my comments and easy-to-read formatting.
If a .css or .js file is only needed on a few pages, I only include a compressed version of that file where needed. (and I use the compressed version here too...) But overall, this has proved to be a good strategy for minimizing website overhead - and increasing speed.
TOOLS TO BOOKMARK:
Speed Tests:
http://tools.pingdom.com/fpt/
http://www.selfseo.com/website_speed_test.php
Compression Tools:
http://javascriptcompressor.com/
http://www.cssdrive.com/index.php/main/csscompressor/
Wednesday, May 12, 2010
Finishing up My Own Software
I've never done this before... but on 15 Mar I will have completed my first software for resale.
I have built software for myself before... including a CMS and E-Commerce application. But this is the first time I have completed a software package for public resale. It is a lot of fun.
This bit of software wraps a set of web services and includes data obtained from my personal research. It allows (through IP-detection) the discovery of the visitor's location, timezone, and allows for conversion of date/time to that location's timezone - using that country's preferred date/time displays.
I have built software for myself before... including a CMS and E-Commerce application. But this is the first time I have completed a software package for public resale. It is a lot of fun.
This bit of software wraps a set of web services and includes data obtained from my personal research. It allows (through IP-detection) the discovery of the visitor's location, timezone, and allows for conversion of date/time to that location's timezone - using that country's preferred date/time displays.
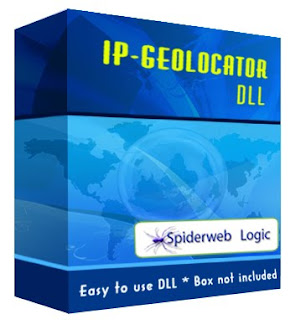
Friday, April 9, 2010
link rel="image_src" - whats this for?
I saw this in a page and was trying to figure out what this was used for..
[link rel="image_src" href="linktoimage.jpg" /]
Basically, when you submit/share this to Facebook , this helps Facebook determine which image to put next to the link. If this is not present, Facebook tries to put in the first image it finds on the page, which may not be the best one to represent your site. (NOTE: Facebook also uses your meta title and meta description tags.)
I am hoping that someday that search engines will use this to put thumbnails of this image in their results display... But oh well. Until then, it will help with social sharing. Since Facebook is such a large resource, this is currently an important thing to consider.
Resources:
Optimizing your site for Facebook
Enhanced Social Share
Facebook Meta Tags
[link rel="image_src" href="linktoimage.jpg" /]
Basically, when you submit/share this to Facebook , this helps Facebook determine which image to put next to the link. If this is not present, Facebook tries to put in the first image it finds on the page, which may not be the best one to represent your site. (NOTE: Facebook also uses your meta title and meta description tags.)
I am hoping that someday that search engines will use this to put thumbnails of this image in their results display... But oh well. Until then, it will help with social sharing. Since Facebook is such a large resource, this is currently an important thing to consider.
Resources:
Optimizing your site for Facebook
Enhanced Social Share
Facebook Meta Tags
Thursday, April 1, 2010
Marching Ants - CSS
I just saw a really cool way to do the marching ants.. Check this out:
http://sunpig.com/martin/archives/2010/02/10/marching-ants-in-css.html
Thought I would pass this along... too cool.
I am always looking for better ways of doing things, and I liked how he used CSS and animated gifs to get the affect...
similar one (though I like the first one better):
http://www.serafinistudios.com/blog/archives/2003/10/29/how-to-add-marching-ants-photoshop-selection-style-to-your-links/
I even integrated this into my CMS - so when people hover over a wigit - they can easily see what sections they can edit.
I had to share
http://sunpig.com/martin/archives/2010/02/10/marching-ants-in-css.html
Thought I would pass this along... too cool.
I am always looking for better ways of doing things, and I liked how he used CSS and animated gifs to get the affect...
similar one (though I like the first one better):
http://www.serafinistudios.com/blog/archives/2003/10/29/how-to-add-marching-ants-photoshop-selection-style-to-your-links/
I even integrated this into my CMS - so when people hover over a wigit - they can easily see what sections they can edit.
I had to share
Friday, March 19, 2010
Google Products - SEO
I have been working on the SEO for one of my clients - and have had a lot of success working on the SEO for his Google Products listing also... It is surprising how this can grab a little-tapped market.
I simply have a RSS feed of my products in a manner requested by Google, and submit the feed to Google Products database. The feed is dynamic - and changes whenever products change using ASP.Net
However, lately I have enhanced it a bit with better success. I still use the page meta-description for the Google product description, but I use a special field for the Google Product name, so we can tailor it for Google Product searches.
Normally I found it to be best practice to include a good product name (with relevant keywords), followed by the package/product size or weight in parenthesis. The product name should include the keywords AND be user-friendly to read. The description should include keywords AND be a good sales pitch.
I simply have a RSS feed of my products in a manner requested by Google, and submit the feed to Google Products database. The feed is dynamic - and changes whenever products change using ASP.Net
However, lately I have enhanced it a bit with better success. I still use the page meta-description for the Google product description, but I use a special field for the Google Product name, so we can tailor it for Google Product searches.
Normally I found it to be best practice to include a good product name (with relevant keywords), followed by the package/product size or weight in parenthesis. The product name should include the keywords AND be user-friendly to read. The description should include keywords AND be a good sales pitch.
Sunday, February 28, 2010
.htaccess file 301 redirect - to avoid duplicate content
Ya - I'm an ASP.net programmer, but every now and then I do a bit of php or other maitenance..
The other day I had to update a website for SEO. I noticed that this guy had two domains pointed to the same website... and that you can crawl them exactly the same.
Unfortunately, this gets a duplicate content penalty so I had to update the .htaccess file to correct this and do 301 redirects. Here are the lines of code needed to do this if you ever need it:
RewriteCond %{HTTP_HOST} ^^(www\.)?dontneedthis\.com$ [NC]
RewriteRule ^(.*)$ http://www.spiderweblogic.com/$1 [R=301,L]
Of course - change spiderweblogic to your website...
TIP: don't kill any existing .htaccess file. Just append this code towards the top. (You may not see it if you use a ftp program.)
The other day I had to update a website for SEO. I noticed that this guy had two domains pointed to the same website... and that you can crawl them exactly the same.
Unfortunately, this gets a duplicate content penalty so I had to update the .htaccess file to correct this and do 301 redirects. Here are the lines of code needed to do this if you ever need it:
RewriteCond %{HTTP_HOST} ^^(www\.)?dontneedthis\.com$ [NC]
RewriteRule ^(.*)$ http://www.spiderweblogic.com/$1 [R=301,L]
Of course - change spiderweblogic to your website...
TIP: don't kill any existing .htaccess file. Just append this code towards the top. (You may not see it if you use a ftp program.)
Friday, January 15, 2010
Pinging Blogs
For best results after posting blogs...ping them. That way blog search sites know you have updated content.
Here are some free services that ping blogs on request:
http://www.feedping.com/
http://pingomatic.com/
If you roll-your-own blog, you may want to use pingomatic.com - since they are easy to integrate as a back-end service.
Here are some free services that ping blogs on request:
http://www.feedping.com/
http://pingomatic.com/
If you roll-your-own blog, you may want to use pingomatic.com - since they are easy to integrate as a back-end service.
Subscribe to:
Posts (Atom)